Working with companies
We noted that you can also use companies to group customers. We can follow a similar process as we did with segments and tags to show how to list people that belong to a certain company. First we need to know how to create companies and add users to those companies. Then we can look at how to list the people that are associated with that company
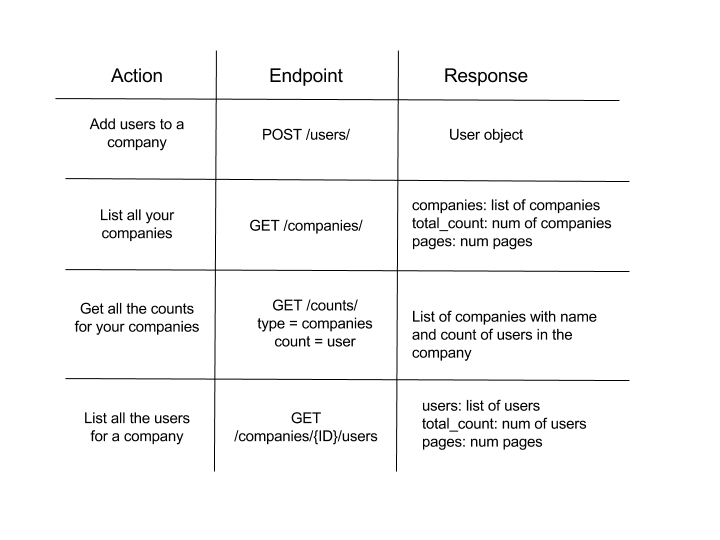
The actions and associated endpoints for using companies
Add people to a company
If you add people to a company that does not exist it will be created. So you can create and update a company all in one go. While you can create a company from the companies endpoint it is easier to simply add users to a company. If the company does not exist then it will be created.
var Intercom = require('intercom-client');
var client = new Intercom.Client({ token: process.env['AT'] });
var cust_id = process.argv[2];
var comp_id = process.argv[3];
var comp_name = process.argv[4];
var customer_data = {
id: cust_id,
companies: [
{
"company_id" : comp_id,
"name" : comp_name
}
]};
client.users.create(customer_data, function (rsp){
console.log(rsp.body)
});
// You can do this multiple times to add more than one user
// Or simply add a list of users in one go
node add_to_company.js '5a03e2d33e69b29660557c9f' '1178' 'Mitch_and_Murray'
{ type: 'user',
id: '4a02c2d33e69b29660557b6d',
user_id: '0',
anonymous: false,
email: '[email protected]',
phone: null,
name: 'Test User',
companies:
{ type: 'company.list',
companies:
[ { type: 'company',
company_id: '1178',
id: '5a047e7c937c888ae37def04',
name: 'Mitch_and_Murray' } ] }
..... (Full user object) .......
tags: { type: 'tag.list', tags: [] },
segments: { type: 'segment.list', segments: [ [Object], [Object] ] },
custom_attributes: {} }
Empty companies in UI and API lists?
If you create a company with no customers associated with it then you will not be able to see it in the UI or list it via the API. You must have someone in the company to be able to list it.
List companies and get user counts
Now that we have created a company by associating users with it we can list our companies and then get the counts associated with those companies.
var Intercom = require('intercom-client');
var client = new Intercom.Client({ token: process.env['AT'] });
client.companies.list(function (rsp){
console.log(rsp.body)
});
// List the companies to get all the companies
> node list_companies.js
{ type: 'company.list',
pages:
{ type: 'pages',
next: null,
page: 1,
per_page: 15,
total_pages: 1 },
companies:
[ { type: 'company',
company_id: '1178',
id: '5a047e7c937c888ae37def04',
app_id: 'fyq3wodw',
name: 'Mitch_and_Murray',
created_at: 1510243964,
updated_at: 1510245247,
last_request_at: 1510245060,
monthly_spend: 0,
session_count: 0,
user_count: 3,
tags: [Object],
segments: [Object],
plan: {},
custom_attributes: [Object] },
{ type: 'company',
company_id: '11',
id: '59945bb33791c186d0f93fbb',
app_id: 'fyq3wodw',
name: 'Hydra2',
created_at: 1502895027,
updated_at: 1505481751,
last_request_at: 1500288932,
monthly_spend: 0,
session_count: 0,
user_count: 2,
tags: [Object],
segments: [Object],
plan: [Object],
custom_attributes: {} },
.... (list of all full company objects) ....
....
....
],
total_count: 10 }
var Intercom = require('intercom-client');
var client = new Intercom.Client({ token: process.env['AT'] });
client.counts.companyUserCounts(function (rsp){
console.log(rsp.body)
});
> node count_companies.js
{ user:
[ { ACME: 0, remote_company_id: '234' },
{ Intercom: 1, remote_company_id: '5' },
{ Test Company: 0, remote_company_id: '9' },
{ Academy: 1, remote_company_id: '10' },
{ Hydra: 1, remote_company_id: 'hydra' },
{ Hydra2: 2, remote_company_id: '11' },
{ Serenity: 1, remote_company_id: '366' },
{ Hydra5: 1, remote_company_id: '79' },
{ Mitch_and_Murray: 3, remote_company_id: '1178' },
{ Acme: 0, remote_company_id: '1121' } ] }
// Remember you can also tag companies
// so you can list them by tag or segment id
client.companies.listBy({ tag_id: 'haven' }, callback);
client.companies.listBy({ segment_id: 'haven' }, callback);
// You can also get the counts for these company tags and segments
client.counts.companyTagCounts(callback);
client.counts.companySegmentCounts(callback);
Empty companies in counts?
Notice that you can see the companies with no users associated with them when you list the companies via the counts endpoint
List all the users in a company
So far we have associated users with companies and been able to list all our companies and even check the number of users associated with these companies. We can now finally list all the users associated with a particular company
var Intercom = require('intercom-client');
var client = new Intercom.Client({ token: process.env['AT'] });
var comp_id = process.argv[2];
// List users in the company using ID (i.e. not company ID)
client.companies.listUsers({ id: comp_id }, function(rsp){
console.log(rsp.body)
});
> node list_company_users.js 5a047e7c937c888ae37def04
# Returns a list of full user objects
{ type: 'user.list',
pages:
{ type: 'pages',
next: null,
page: 1,
per_page: 50,
total_pages: 1 },
users:
[ { type: 'user',
id: '23ca7c87d4c42de1156ef9e4',
user_id: '124',
anonymous: false,
email: '[email protected]',
phone: null,
name: 'bobr',
pseudonym: null,
avatar: [Object],
app_id: 'fyq3wodw',
companies: [Object],
.... (full user object) ...
{ type: 'user',
id: '59ca900e01dc2e62528f13f8',
user_id: '187',
anonymous: false,
email: '[email protected]',
phone: null,
.... (full user object) ...
custom_attributes: [Object] },
{ type: 'user',
id: '5a03e2d33e69b29660557c9f',
user_id: '0',
anonymous: false,
.... (full user object) ...
],
total_count: 3
}
Updated about 5 years ago