Converting visitors and leads to users
Converting visitors and leads to users
Once you understand what visitors, leads and users mean in Intercom you can start using them in your own workflows. We will work through some simple examples here to show you how you can convert visitors and leads to users.
Use a TEST app?
If you want to try out some of these actions but are afraid to do this on your main app you can always create a TEST Intercom app from your current app. Then you can try out any actions without risking compromising your live data. We show you how to setup a TEST app as part of the OAuth flow here. So you can take a look at that and see if your want to set it up and use it for testing. Remember, you don't need OAuth, its just the TEST section which you may find helpful.
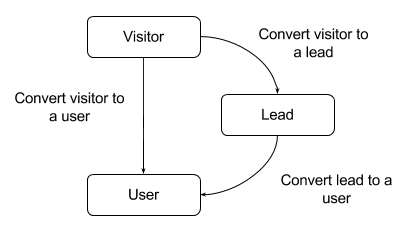
The actions you can take to convert visitors, leads and users
Getting your visitor ID
As we noted earlier, visitors are not stored in the Intercom UI in the same way leads or users are. As a result, you cannot simply look through your visitors to retrieve their IDs. Instead you need to use the Intercom JS code to get a visitor's ID when they are browsing through your site.
To show how you can do this we created a simple interactive example using Codepen. In the example below you put your own App ID in the Codepen editor as described on the page:
Live visitor example
You can find the interactive Codepen example here where you can add your own App ID and start testing how to get your visitor ID and convert users via the Messenger.
Note here that the user or lead you create is not converted from any pre-existing user. To show you how to convert between these entities we can use the Intercom API in the below worked examples.
Converting visitors to leads
Now that you can get your visitor ID via the Intercom JS code you can convert that to a lead at any time on your pipeline process. For more info on the API operation see our API reference for converting visitors
var Intercom = require('intercom-client');
var client = new Intercom.Client({ token: process.env['AT'] });
// This is the Visitor ID you retrieved via JS code
var vid = process.argv[2];
// This will create a lead from the vid
var conversion = {
visitor: { user_id: vid },
type: "lead"
};
client.visitors.convert(conversion,
function (rsp) {
console.log(rsp.body.pseudonym)
});
> node convert_lead.js '4b34499c-9b11-4546-bf77-e556a6503a80'
# This will return pseudonym of the new lead created
Blue Rocket from Dublin
Create new visitor ID
Remember you can generate a new visitor ID in the example Codepen using the "Sign Out" button. This ensures there is no carry over between conversations but can also be used to generate a new visitor ID so you can test out the convert action multiple times (the visitor is deleted once they are converted so you will need to get a new visitor ID to perform another test)
If you run the above code the visitor will be deleted and a new lead will be created.
Converting visitors to users
You can also convert a visitor directly to a user. Again, this all depends on your particular pipeline requirements. You may have certain criteria that you mean you want to convert straight to a user such as they subscribe to a product without ever communicating to you via the Messenger.
var Intercom = require('intercom-client');
var client = new Intercom.Client({ token: process.env['AT'] });
var vid = process.argv[2];
var usr_email = process.argv[3];
// Convert visitors into Users
var conversion = {
visitor: { user_id: vid },
user: { email: usr_email },
type: "user"
};
// Return the body of the user
client.visitors.convert(conversion,
function (rsp) {
console.log(rsp.body)
});
node convert_user.js 'd5be9d48-0f0f-4454-9096-c90f4d55c4fb' 'test_convert@example.com'
# Will return the user body
{ type: 'user',
id: '59ecb5a0e541ecaa500e299e2',
user_id: null,
anonymous: false,
email: 'test_convert@example.com',
phone: null,
name: null,
pseudonym: 'Cyan Sailboat from Dublin',
avatar: { type: 'avatar', image_url: null },
app_id: 'fyq3wodw',
companies: { type: 'company.list', companies: [] },
....
}
Convert or merge?
If no user exists then the visitor convert action will create a brand new user. Alternatively, if you want to merge a visitor to one of your existing users then you can provide an identifier (e.g. user email or user ID) and the visitor will be merged with the user. In both cases the visitor will be deleted.
Converting leads to users
In the same way you can convert visitors to users you can also convert leads to users. Again, this will be dependant on your particular pipeline configuration so we will show you how to do this here and you can choose when to do it in your flow.
You can also checkout our API reference page here if you would like to see more detail on how to convert between leads and users.
Create a new lead
Remember, to create a new lead you just need to say hello in the Messenger on your site. A new lead will automatically be created on your app. You can get the leads identifier and use it in the below example.
If you run the following code you will be able to convert your leads to new users or merge them with existing users. Similar to visitors, if a user exists you will merge the leads information with that user. If the user does not exist then you will create a new user. In both cases the lead is deleted.
You always need to specify a user identifier
Even if your user does not exist you will need to specify an identifier, e.g. email or user_id, for that user. If there is no user one will be created with that provided identifier.
var Intercom = require('intercom-client');
var client = new Intercom.Client({ token: process.env['AT'] });
var lead_id = process.argv[2];
var usr_id = process.argv[3];
// Convert Leads into Users
var conversion = {
// Remeber to us the URL ID to identify leads
contact: { id: lead_id},
user: { user_id: usr_id }
};
// Return the body of the user
client.leads.convert(conversion,
function (rsp) {
console.log(rsp.body)
});
node lead_user.js '59fc5cc01dbd216027c1c136' 'test_lead_user@example.com'
Updated over 7 years ago