Android
Configuring Intercom for Mobile on Android
Track who your users are and what they do in your mobile app and customize the Intercom Messenger. Here’s how to configure Intercom for Android:
Update a user
You can send any data you like to Intercom from standard user attributes that are common to all Intercom users to custom user attributes that are unique to your app.
The complete list of standard user attributes that can be updated are described in the UserAttributes object. Standard user attributes such as a user's name or email address can be updated by calling:
UserAttributes userAttributes = new UserAttributes.Builder()
.withName("Bob")
.withEmail("bob@example.com")
.build();
Intercom.client().updateUser(userAttributes);
Typically our customers see a lot of value in sending custom data that relates to customer development, such as price plan, value of purchases, etc. Custom user attributes can be created and modified by calling withCustomAttribute(key, value) on the UserAttributes object.
UserAttributes userAttributes = new UserAttributes.Builder()
.withCustomAttribute("paid_subscriber", "Yes")
.withCustomAttribute("monthly_spend", 155.5)
.withCustomAttribute("team_mates", 3)
.build();
Intercom.client().updateUser(userAttributes);
You don’t have to create attributes in Intercom beforehand. If a custom attribute hasn't been seen before, it will be created for you automatically.
You can also set company data on your user with the Company object, like:
Company company = new Company.Builder()
.withName("My Company")
.withCompanyId("abc1234")
.build();
UserAttributes userAttributes = new UserAttributes.Builder()
.withCompany(company)
.build();
Intercom.client().updateUser(userAttributes);
- companyId is a required field for adding or modifying a company.
- The Company object describes all the standard attributes you can modify.
Submit an event
You can log events in Intercom that record what users do in your app and when they do it. For example, you could record the item a user ordered from your mobile app, and when they ordered it.
Map<String, Object> eventData = new HashMap<>();
eventData.put("order_date", "1392036272");
eventData.put("stripe_invoice", "38572984");
Intercom.client().logEvent("sent_invitation", eventData);
You’ll find more details about how events work and how to submit them here.
Customize the Intercom Messenger
We definitely recommend that you customize the Intercom Messenger so that it feels completely at home on your product, site or mobile app. Here’s how:
- Select the color and language of the Messenger and how personalize your profiles.
- Follow the below steps to choose how the launcher appears and opens for your users.
Choose how the launcher appears and opens for your users
If you’d like the standard launcher to appear on the bottom right-hand side of your screen, just call:
Intercom.client().setLauncherVisibility(Visibility.VISIBLE);
Create a custom launcher
However, if you’d like the Messenger to open from another location in your mobile app, you can create a custom launcher. This allows you to specify a button, link or element that opens the Messenger. For example, you can trigger the launcher to open when a customer clicks on your ‘Help and Support’ button.
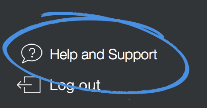
If you have a custom launcher, you can call Intercom.client().displayMessenger();
and we’ll ensure the Messenger opens in the best place for each user. For example:
- If a user has one unread conversation, we open that conversation.
- If a user has no conversations, we open the composer.
- If a user has more than one unread conversation, we open the conversations list.
- If a user has no unread conversations, we open the last screen they were on when they closed it.
Show your user’s unread message count
Now you can show how many unread conversations your user has on your custom launcher. Even if a user dismisses a notification, they’ll still have a persistent indicator of unread conversations.
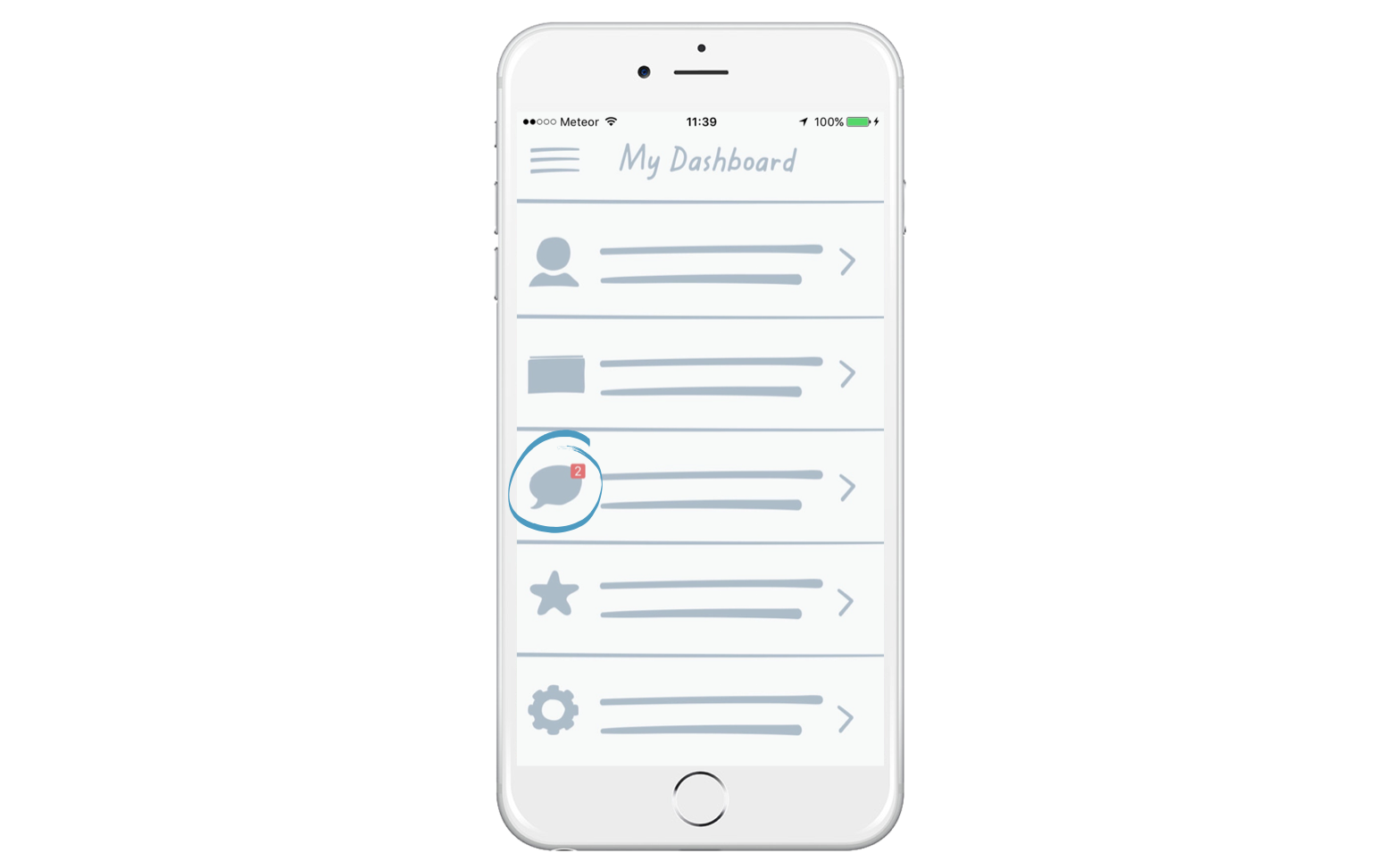
Just grab the current count with this method:
Intercom.client().getUnreadConversationCount();
Then, start listening for updates using:
Intercom.client().addUnreadConversationCountListener(listener);
Temporarily hide notifications
You can prevent in app messages from popping up in certain parts of your app by calling:
Intercom.client().setInAppMessageVisibility(Visibility.GONE);
You can hide the Intercom Messenger in your app by calling:
Intercom.client().hideMessenger();
Articles Help Center
From version 4.1.7 of the Android Messenger we support opening up your Educate Help Center.
https://docs.intercom.com/educating-your-customers .
To open up the Help Center simply call Intercom.client().displayHelpCenter()
and we will display an Activity with your Help Center content in it.
Make sure Help Center is turned on
If you don't have Help Center enabled in your Intercom settings the method
displayHelpCenter
will log an error and not open the Activity. To enable your Help Center please go here and click the "Turn On Help Center" button.
What's next?
Now that you have Intercom configured you can:
- Enable Identity Verification.
- Configure Android Push Notifications for GCM or FCM.
Updated over 6 years ago