iOS
iOS push notifications setup
This article explains how to enable push notifications in Intercom for iOS. If you are new to push notifications in iOS, check out this page first.
To enable push notifications in Intercom you first need to create a PEM file of your Apple Push Certificate and upload it to Intercom (we support both production and development PEM files).
Step 1: Create App ID and APNS SSL Certificate
You can skip this step if you have already configured your iOS app to support the Apple Push Notification Service (APNS).
Login to your Apple developer account. Edit the app id for your app and tick the push notification option to configure the certificate as shown below.
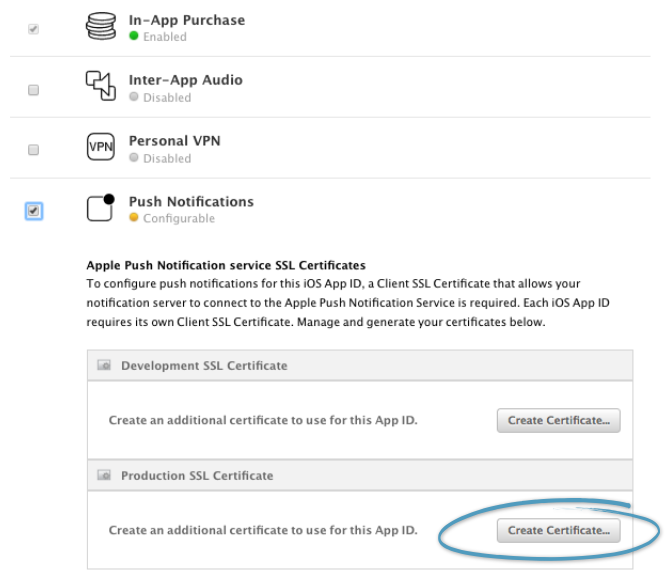
Follow the steps as described (Select type, create a Certificate Signing Request, Generate and Download the certificate). Double click the certificate (.cer file) to import it into your keychain.
Step 2: Export your APNS SSL Certificate
First, launch "Keychain Access" on your Mac and find the iOS push certificate for your app. This will be within the “My Certificates" category. You will know it is the correct one as it will start with “Apple Push Services:" followed by the bundle id of your app.
Next, select both the certificate and the private key it contains, then right click and choose to export. Make sure that the file format is set to "Personal Information Exchange (.p12)" and save it to your Desktop.
Important
Do not enter a password when prompted for protecting the export.
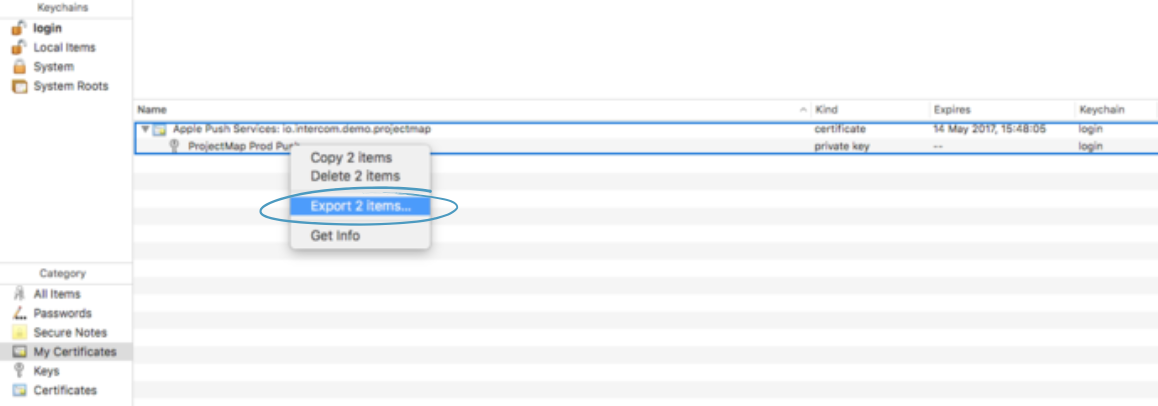
Next you will need to create a PEM file from this newly exported .p12. You can do this easily in the terminal by moving into your Desktop directory or wherever you saved the .p12 file. Then enter this command:
openssl pkcs12 -in Certificates.p12 -out Certificates.pem -nodes
You should now have the PEM file created on your Desktop.
Step 3: Upload PEM file to Intercom
Go to your app's settings and select Intercom for iOS. You'll notice the "Enable Push Notifications" section where you'll be able to upload your newly created PEM file.
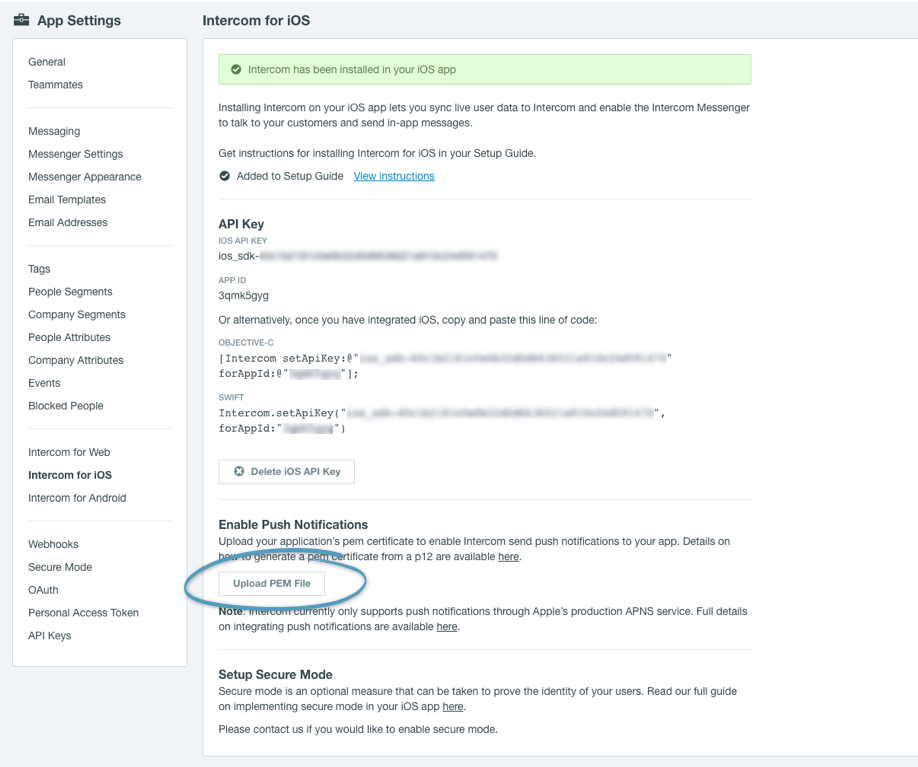
Debug and Release Support
Intercom supports push notifications on both Debug and Release builds 😉
Step 4: Download the Provisioning Profile
You can skip this step if you have already configured your iOS app to support the Apple Push Notification Service (APNS).
- Login to your Apple developer account. Create an iOS Provisioning Profile for the Production environment.
- Select the App ID you created in Step 1 and generate / download the profile.
- Double click the .mobileprovision file to import it into Xcode.
- In the Xcode Build Settings for your target, select the correct Code Signing Identify and Provisioning Profile.
- Install a build of the app on your iOS device.
Step 5: Register with APNS and register Device Tokens
As usual, you should also register with the Apple Push Notification Service (APNS) by adding this code to applicationDidBecomeActive if you haven't already:
- (void)applicationDidBecomeActive:(UIApplication *)application {
[application registerUserNotificationSettings:
[UIUserNotificationSettings settingsForTypes:(UIUserNotificationTypeBadge | UIUserNotificationTypeSound | UIUserNotificationTypeAlert)
categories:nil]];
[application registerForRemoteNotifications];
}
func applicationDidBecomeActive(_ application: UIApplication) {
let settings = UIUserNotificationSettings(types: [.badge, .sound, .alert], categories: nil)
application.registerUserNotificationSettings(settings)
application.registerForRemoteNotifications()
}
In order to enable your users receive push notifications from Intercom via Intercom for iOS, you must register the device token of your user.
- (void)application:(UIApplication *)application didRegisterForRemoteNotificationsWithDeviceToken:(NSData *)deviceToken {
[Intercom setDeviceToken:deviceToken];
}
func application(_ application: UIApplication, didRegisterForRemoteNotificationsWithDeviceToken deviceToken: Data) {
Intercom.setDeviceToken(deviceToken)
}
At this stage you should make sure that you have enabled the Push Notifications capability in Xcode.
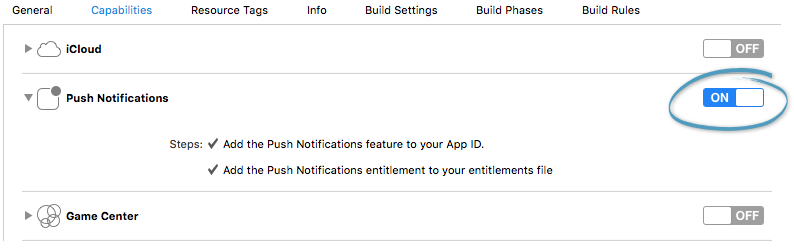
Step 6: Handling Intercom Push Notifications
When your app receives a push notification Intercom for iOS checks to see if it is an Intercom push notification and opens the message if required. You do not need to implement any additional code in order to launch Intercom's UI once you have followed the instructions in step 5 above. To do this we safely swizzle the public methods in UIApplicationDelegate
that handle receiving push notifications. We do not use any private APIs to do this.
Manually handle push notifications (Optional)
Optionally, you may disable the swizzling for push notifications by setting IntercomAutoIntegratePushNotifications
to NO
in your app's Info.plist
. You will then need to handle Intercom push notifications as shown here:
- (void)application:(UIApplication *)application didReceiveRemoteNotification:(NSDictionary *)userInfo fetchCompletionHandler:(nonnull void (^)(UIBackgroundFetchResult))completionHandler {
if ([Intercom isIntercomPushNotification:userInfo]) {
[Intercom handleIntercomPushNotification:userInfo];
}
completionHandler(UIBackgroundFetchResultNoData);
}
func application(_ application: UIApplication, didReceiveRemoteNotification userInfo: [AnyHashable : Any], fetchCompletionHandler completionHandler: @escaping (UIBackgroundFetchResult) -> Void) {
if (Intercom.isIntercomPushNotification(userInfo)) {
Intercom.handlePushNotification(userInfo)
}
completionHandler(.noData);
}
Note: it is only necessary to do this if you decide to disable the automatic push notification handling.
Step 7: Testing Intercom Push Notifications
You can easily test if push notifications are working properly in your app. Just send a manual message to the app user via Intercom.
Badge Values
Intercom never changes the badge value of your app. Thus we can ensure that whatever badge value you're managing in your app, we don't alter in any way.
Troubleshooting
If you are having trouble getting push notifications to work in your app, here's a list of things you should check:
- Ensure you ticked the box 'Send a push notification' when you send a manual message.
- When your push-enabled app registers for push notifications for the first time on a device, iOS asks the user if they wish to receive notifications for that app. So please verify that when you launch your app for the first time iOS shows you this alert view. You should tap OK in this case to allow iOS to send you push notifications.
- Do you get a token from APNS? If you put a breakpoint into the application:didRegisterForRemoteNotificationsWithDeviceToken: delegate call, you should get a token shortly after your app launches.
- Did you generate and upload the PEM file correctly? Make sure you downloaded the productionAPNS SLL certificate. Also, ensure you exported both the certificate and private key, and you created the p12 file with an empty password. Double-check that you uploaded the PEM file to the correct Intercom app (TEST vs. Production).
- You can find more technical information and troubleshooting steps in the Apple iOS Developer Library.
And as always, you can contact us via Intercom. We're always here to help 😀
Updated almost 5 years ago