Adding companies
Once you have some users setup in Intercom you can start grouping them using the Intercom companies feature. This allows you to more easily identify and communicate with specific groups of people.
Companies, like tags and custom attributes, are also part of the user model. This makes it easier to access, group and search for data on your users. For more information on companies, and some use case examples, please refer to the Intercom docs.
Creating a company
Companies can exist without users in Intercom but they will not appear in the system. Instead, we can easily create companies by adding them to users. If the company already exists the user will be added to that company. However, if it does not already exist then the company will be created and the user will be added to it.
In this tutorial we'll create companies by adding them to our users. The first step we need to do is to find a valid user to which we can add a company. In a simple format it would look like this:
def create_companies(comp_name, comp_id)
#First check if the user exists,
#It will throw an exception if it fails to find a user
user = UserTasks.new()
usr = user.find_user(criteria)
#Create a hash to pass through the company data
company = {
:company_id => comp_id,
:name => comp_name,
}
usr.companies = ([company])
@@intercom.users.save(usr)
end
require './intercom_client'
require './user_tasks'
class CompanyTasks < IntercomClient
def initialize()
end
def create_companies(comp_name, comp_id, criteria)
#First check if the user exists,
#It will throw an exception if it fails to find a user
user = UserTasks.new()
usr = user.find_user(criteria)
#Create a hash to pass through the company data
company = {
:company_id => comp_id,
:name => comp_name,
}
usr.companies = ([company])
@@intercom.users.save(usr)
end
end
You should then be able to create a company and see this information via the looking at the companies feature in the web app and the user model itself:
> require './test.rb'
> intercom = IntercomClient.new('<ACCESS TOKEN>');
> comp = CompanyTasks.new
> usr = comp.create_companies("PhilosophyCorp", "1", "[email protected]")
> usr.companies
=> [#<Intercom::Company:0x000000031beb50 @changed_fields=#<Set: {}>, @company_id="1", @custom_attributes={}, @id="5746cdf0b7f5891ac3000079", @name="PhilosophyCorp">]
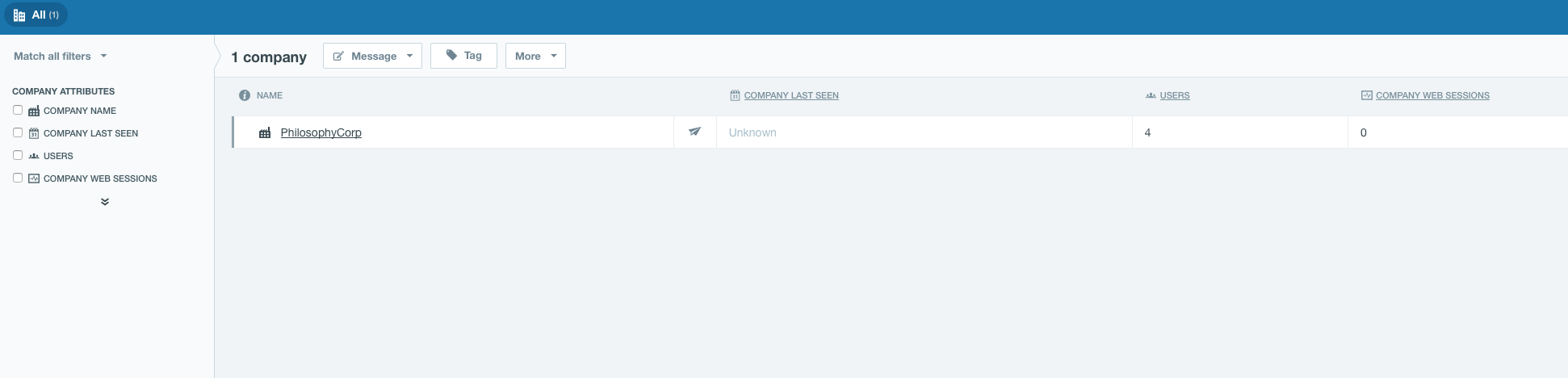
Company Info
Creating a company with custom attributes
Just like a user, a company can also have custom attributes. These may be specific to your business. They could, for example, be a parent or subsidiary company and you may want to store this info when creating that company with a user. Similarly, you may want to store info such as the company size, location or business area.
You can do all of this via company custom attributes. Let's leave this parameter optional so that we can easily create companies without these attributes if we don't want to add them:
def create_companies(comp_name, comp_id, criteria, custom_data=false)
#First check if the user exists,
#It will throw an exception if it fails to find a user
user = UserTasks.new()
usr = user.find_user(criteria)
#Create a hash to pass through the company data
company = {
:company_id => comp_id,
:name => comp_name,
}
#Check for custom attributes
if custom_data
company[:custom_attributes] = custom_data
end
usr.companies = ([company])
@@intercom.users.save(usr)
end
require './intercom_client'
require './user_tasks'
class CompanyTasks < IntercomClient
def initialize()
end
def create_companies(comp_name, comp_id, criteria, custom_data=false)
#First check if the user exists,
#It will throw an exception if it fails to find a user
user = UserTasks.new()
usr = user.find_user(criteria)
#Create a hash to pass through the company data
company = {
:company_id => comp_id,
:name => comp_name,
}
#Check for custom attributes
if custom_data
company[:custom_attributes] = custom_data
end
usr.companies = ([company])
@@intercom.users.save(usr)
end
end
Then you can add some custom info to your companies as follows:
> usr = comp.create_companies("PhilosophyLtd", "2", "[email protected]",{:employees=>"1", :founded=>"1913"})
> usr.companies
=> [#<Intercom::Company:0x00000002ed7450 @changed_fields=#<Set: {}>, @company_id="2", @custom_attributes={}, @id="574706f566574dad3700002e", @name="PhilosophyLtd">]

Company Custom Attributes from User View

Company Custom Attributes from Company View
Finding companies
Now that we have created a company we may want to search for it using either the name, the company ID or the auto created internal ID. To make it easier to search let's make it so you can enter any of these criteria into the search and use the exceptions returned when no company is found to iterate through each option - just like we did with searching for a user:
def find_companies(criteria)
begin
#Use exceptions to check other search criteria
@@intercom.companies.find(:id => criteria)
rescue Intercom::ResourceNotFound
begin
#Check for users via company id if we receive not found error
@@intercom.companies.find(:company_id=> criteria)
rescue Intercom::ResourceNotFound
#Check for users via company name
@@intercom.companies.find(:name => criteria)
end
end
end
require './intercom_client'
require './user_tasks'
class CompanyTasks < IntercomClient
def initialize()
end
def create_companies(comp_name, comp_id, criteria, custom_data=false)
#First check if the user exists,
#It will throw an exception if it fails to find a user
user = UserTasks.new()
usr = user.find_user(criteria)
#Create a hash to pass through the company data
company = {
:company_id => comp_id,
:name => comp_name,
}
#Check for custom attributes
if custom_data
company[:custom_attributes] = custom_data
end
usr.companies = ([company])
@@intercom.users.save(usr)
end
def find_companies(criteria)
begin
#Use exceptions to check other search criteria
@@intercom.companies.find(:id => criteria)
rescue Intercom::ResourceNotFound
begin
#Check for users via company id if we receive not found error
@@intercom.companies.find(:company_id=> criteria)
rescue Intercom::ResourceNotFound
#Check for users via company name
@@intercom.companies.find(:name => criteria)
end
end
end
end
Now let's search for one of the companies we created previously:
> comp.find_companies("PhilosophyLtd");
> company = comp.find_companies("PhilosophyLtd");
> company
=> #<Intercom::Company:0x00000001176be0
@app_id="ja43hiec",
@changed_fields=#<Set: {}>,
@company_id="2",
@created_at=1464272629,
@custom_attributes={"employees"=>"1", "founded"=>"1913"},
@id="574706f566574dad3700002e",
@monthly_spend=0,
@name="PhilosophyLtd",
@plan={},
@segments=[],
@session_count=0,
@tags=[],
@updated_at=1464273049,
@user_count=1>
> company = comp.find_companies(1);
> company
=> #<Intercom::Company:0x00000001b75ad8
@app_id="ja43hiec",
@changed_fields=#<Set: {}>,
@company_id="1",
@created_at=1464258032,
@custom_attributes={},
@id="5746cdf0b7f5891ac3000079",
@monthly_spend=0,
@name="PhilosophyCorp",
@plan={},
@segments=[],
@session_count=0,
@tags=[],
@updated_at=1464273255,
@user_count=1>
Listing companies
If you have lots of companies in your app, you may want to easily list them rather than viewing them in the web app. We can do this by iterating through the companies list.
Since there may be custom attributes, let's allow you to choose what you want to see - whether that's simply a quick list of all the companies with their names and IDs, or a full list of all names, IDs and any custom attributes.
def list_companies(*attribs)
if attribs
@@intercom.companies.all.each do |company|
puts "#{company.name}:#{company.company_id} "
attribs.each {|attrib| puts "- #{attrib}:#{company.custom_attributes[attrib]}"}
end
else
@@intercom.companies.all.map {|company| puts "Company Name: #{company.name}, Company ID: #{company.company_id}" }
end
end
require './intercom_client'
require './user_tasks'
class CompanyTasks < IntercomClient
def initialize()
end
def create_companies(comp_name, comp_id, criteria, custom_data=false)
#First check if the user exists,
#It will throw an exception if it fails to find a user
user = UserTasks.new()
usr = user.find_user(criteria)
#Create a hash to pass through the company data
company = {
:company_id => comp_id,
:name => comp_name,
}
#Check for custom attributes
if custom_data
company[:custom_attributes] = custom_data
end
usr.companies = ([company])
@@intercom.users.save(usr)
end
def find_companies(criteria)
begin
#Use exceptions to check other search criteria
@@intercom.companies.find(:id => criteria)
rescue Intercom::ResourceNotFound
begin
#Check for users via company id if we receive not found error
@@intercom.companies.find(:company_id=> criteria)
rescue Intercom::ResourceNotFound
#Check for users via company name
@@intercom.companies.find(:name => criteria)
end
end
end
def list_companies(*attribs)
if attribs
@@intercom.companies.all.each do |company|
puts "#{company.name}:#{company.company_id} "
attribs.each {|attrib| puts "- #{attrib}:#{company.custom_attributes[attrib]}"}
end
else
@@intercom.companies.all.map {|company| puts "Company Name: #{company.name}, Company ID: #{company.company_id}" }
end
end
end
> comp.list_companies();
PhilosophyLtd:2
PhilosophyCorp:1
> comp.list_companies("employees", "founded");
PhilosophyLtd:2
- employees:1
- founded:1913
PhilosophyCorp:1
- employees:
- founded:
Listing a company's users
Instead of listing companies, you may want to list the users which are linked to a particular company. Again, lets give the option here of listing all the users via one attribute such as email or user_id or the option to return the list of users so that it can be iterated on to perform custom operations.
def list_company_users(criteria, attrib=false)
comp = find_companies(criteria)
users_list = @@intercom.companies.users(comp.id)
#Show specific attrib for each user if specified
if attrib
users_list.each {|usr| puts usr.send(attrib.to_sym)}
else
#Just return the list to allow user perform custom actions
return(users_list)
end
end
require './intercom_client'
require './user_tasks'
class CompanyTasks < IntercomClient
def initialize()
end
def create_companies(comp_name, comp_id, criteria, custom_data=false)
#First check if the user exists,
#It will throw an exception if it fails to find a user
user = UserTasks.new()
usr = user.find_user(criteria)
#Create a hash to pass through the company data
company = {
:company_id => comp_id,
:name => comp_name,
}
#Check for custom attributes
if custom_data
company[:custom_attributes] = custom_data
end
usr.companies = ([company])
@@intercom.users.save(usr)
end
def find_companies(criteria)
begin
#Use exceptions to check other search criteria
@@intercom.companies.find(:id => criteria)
rescue Intercom::ResourceNotFound
begin
#Check for users via company id if we receive not found error
@@intercom.companies.find(:company_id=> criteria)
rescue Intercom::ResourceNotFound
#Check for users via company name
@@intercom.companies.find(:name => criteria)
end
end
end
def list_companies(*attribs)
if attribs
@@intercom.companies.all.each do |company|
puts "#{company.name}:#{company.company_id} "
attribs.each {|attrib| puts "- #{attrib}:#{company.custom_attributes[attrib]}"}
end
else
@@intercom.companies.all.map {|company| puts "Company Name: #{company.name}, Company ID: #{company.company_id}" }
end
end
def list_company_users(criteria, attrib=false)
comp = find_companies(criteria)
users_list = @@intercom.companies.users(comp.id)
#Show specific attrib for each user if specified
if attrib
users_list.each {|usr| puts usr.send(attrib.to_sym)}
else
#Just return the list to allow user perform custom actions
return(users_list)
end
end
end
> usr_list = comp.list_company_users(1);
> usr_list.each {|user| puts user.email};
[email protected]
> comp.list_company_users(2, "email");
[email protected]
You should now be able to group your users by companies and also search for and manage your users based on this data.
Updated over 7 years ago