Using segments
Segments
Segments are groups of users who match certain criteria, specified by you. Segments differ from tags in that they are automatically created whenever a user matches these criteria, whereas tags are added to users manually. You can find more information on segments here which will help you understand how to configure the filters via Intercom.
The API supports fetching the list of segments created in your App, however creating and managing segments must be done through Intercom.
Listing existing segments
We can list all of our segments first to see what segments are setup by default. We can create a simple segment_tasks.rb file as follows to list our segments:
require './intercom_client'
class SegmentTasks < IntercomClient
def initialize()
#Override initialize in IntercomClient
end
def list_segments()
@@intercom.segments.all.each {|segment| puts "id: #{segment.id} name: #{segment.name}"}
end
end
Now let's list our segments:
> require './segment_tasks'
=> true
> intercom = IntercomClient.new('<YOUR ACCESS TOKEN>')
=> #<IntercomClient:0x000000019a5fa0>
> seg = SegmentTasks.new
=> #<SegmentTasks:0x00000001758ce8>
> seg.list_segments
id: 570e30532b1f6e8694000092 name: New
id: 570e30532b1f6e8694000093 name: Active
id: 570e30532b1f6e8694000094 name: Slipping Away
You can see there are IDs associated with each of the default segments so let's get the segments associated with these IDs so we can look at them more closely.
Retrieving a segment object
We can create a simple method to return a segment object given the segment ID:
def get_segment(seg_id)
@@intercom.segments.find(:id => seg_id)
end
require './intercom_client'
class SegmentTasks < IntercomClient
def initialize()
#Override initialize in IntercomClient
end
def get_segment(seg_id)
@@intercom.segments.find(:id => seg_id)
end
def list_segments()
@@intercom.segments.all.each {|segment| puts "id: #{segment.id} name: #{segment.name}"}
end
end
We can get things like the segment name from the returned segment object:
> test = seg.get_segment("570e30532b1f6e8694000092")
> test.name
=> "New"
Note that these segment objects do not contain any user information, though segments are actually part of the user models. What we can do to make this more useful is get the individual segments and then do a simple iteration over your users to see which segments they are on.
Finding users by segment
We can create a new segment and then list it to see if it contains users. Remember we need to do this via the Intercom web app as outlined in the documentation. Let's create a segment which looks for any user_id which contains 1 (which should be all our users):
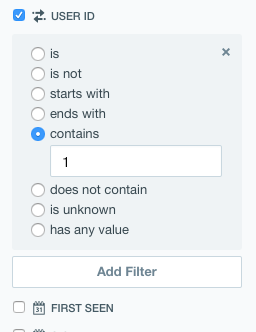
Now let's list all of our segments:
> seg.list_segments
id: 570e30532b1f6e8694000093 name: Active
id: 570e30532b1f6e8694000092 name: New
id: 570e30532b1f6e8694000094 name: Slipping Away
id: 5720e6ac7175427af100001b name: user_id with 1
Let's see if we can list all of the users in our new segment. We can iterate through our users manually and search for the segment. Alternatively, we can use the "list users by segments" feature already available via the API. Both options are shown below:
def get_segment(seg_id)
#Let's check for the segment first.
#If it is not there then this will throw an exception
@@intercom.segments.find(:id => seg_id)
segs = @@intercom.get("/users", segment_id: seg_id)
segs['users'].each {|usrs| puts usrs['email']}
#Alternatively you could iterate through the users list manually
#@@intercom.users.all.map do |user|
# user.segments.each {|val| puts user.email if val.id.include?(seg_id)}
#end
end
require './intercom_client'
class SegmentTasks < IntercomClient
def initialize()
#Override initialize in IntercomClient
end
def get_segment(seg_id)
#Let's check for the segment first.
#If it is not there then this will throw an exception
@@intercom.segments.find(:id => seg_id)
segs = @@intercom.get("/users", segment_id: seg_id)
segs['users'].each {|usrs| puts usrs['email']}
#Alternatively you could iterate through the users list manually
#@@intercom.users.all.map do |user|
# user.segments.each {|val| puts user.email if val.id.include?(seg_id)}
#end
end
def list_segments()
@@intercom.segments.all.each {|segment| puts "id: #{segment.id} name: #{segment.name}"}
end
end
Now we can list our segments and then list the users associated with these segments:
> require './segment_tasks'
=> true
> intercom = IntercomClient.new('<YOUR ACCESS TOKEN>')
=> #<IntercomClient:0x00000002b13eb8>
> seg = SegmentTasks.new
=> #<SegmentTasks:0x00000002aa2ad8>
> seg.list_segments
id: 570e30532b1f6e8694000093 name: Active
id: 570e30532b1f6e8694000092 name: New
id: 570e30532b1f6e8694000094 name: Slipping Away
id: 5721e422f0d93eaaf500001a name: email_contains_eg
id: 5720e6ac7175427af100001b name: user_id with 1
> seg.get_segment("570e30532b1f6e8694000093")
> ##No users in this segment
> seg.get_segment("5720e6ac7175427af100001b")
[email protected]
[email protected]
[email protected]
[email protected]
[email protected]
[email protected]
Updated almost 7 years ago